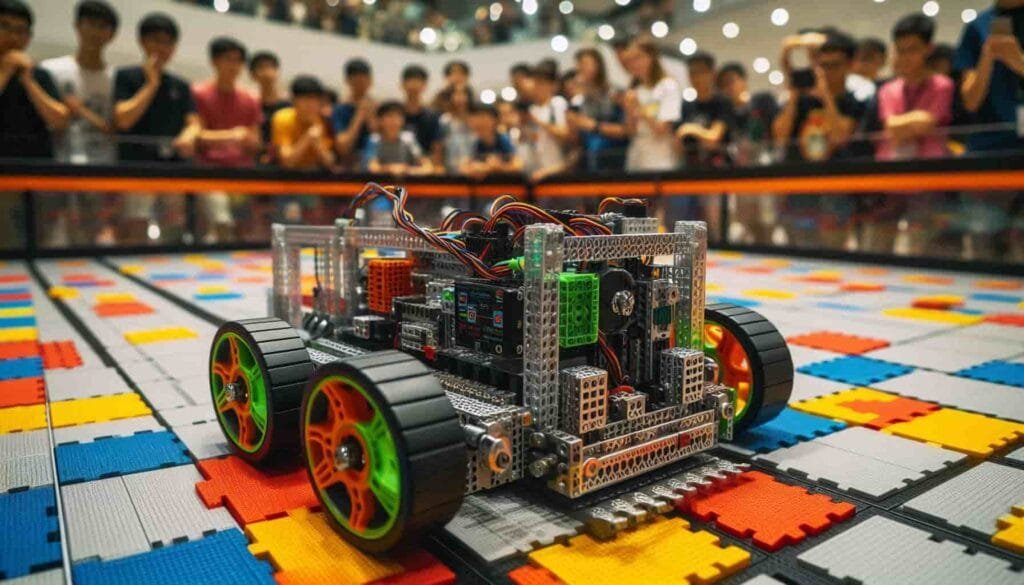
Imagine trying to operate an automobile without influence over its speed. frustrating, right? Motor speed control is also rather crucial in robots. Knowing how to set motor speed c vex v5 system is essential whether your project is a classroom or a competition robot. From the foundations to sophisticated methods, this tutorial will walk you through what you need to know so you can have your robot functioning fault-free.
Understanding VEX V5 Motors
What are VEX V5 Smart Motors?
Usually VEX robots are driven by VEX V5 Smart Motors. These motors have built-in sensors, smart ports, and torque, position, and speed measuring capability unlike your typical DC motors.
Features and Capabilities
- Integrated Encoder: records the motor’s position and speed.
- Programmable Speed: lets one precisely control things with codes.
- Multiple Modes: Add hold, brake, and coast modes for flexible movement.
Why Do Motors Matter?
The control of your robot’s motors determines its performance in great part. Change in speed affects navigation, task efficiency, and even power consumption.
Fundamentals of C Programming for VEX V5
Why Use C in Robotics?
Robotics heavily rely on C for performance and hardware control features. C is applied for VEX V5 using VEXcode Pro V5, an easy IDE made especially for robotics programming.
Getting Started with VEXcode Pro V5
To begin programming:
- Get and run VEXcode Pro V5.
- Get acquainted with the user interface.
- Connect your PC to your Vex V5 Brain.
Setting Up Your Project
Installing and Configuring
Verify your installed libraries and newest firmware. This ensures compatibility and access to all features.
Creating a New Project
- Open VEXcode Pro V5 here.
- Launch a fresh project and choose “C++”.
- Name your project and save it somewhere you will find convenient.
Connecting Hardware
- Outline your motors into the V5 Brain ports.
- Check connections from the VEXcode devices menu.
Introduction to Motor Control in C
The Motor Object
Controlling your motors in VEXcode Pro V5 requires the motor object. This is a simple arrangement:
cpp
Copy code
motor Motor1(PORT1, ratio18_1, false);
Syntax Breakdown
- PORT1: Specifies the motor port.
- ratio18_1: Sets the gear ratio.
- false: Indicates motor rotation direction.
Understanding Motor Speed Parameters
What Is Motor Speed?
Motor speed controls a motor’s rotation speed. Usually found in VEX V5, this is expressed as either RPM or %.
RPM vs Percentage
- RPM (Revolutions Per Minute): exact control for more complex jobs.
- Percentage: For novices, simpler, more understandable control.
Basic Commands to Set Motor Speed
Using motor.spin
Your regular tool for motor control is the motor.spin command:
cpp
Copy code
Motor1.spin(forward, 50, percent);
- forward: Direction of rotation.
- 50: Speed (percentage or RPM).
- percent: Unit of measurement.
Example Code
cpp
Copy code
#include “vex.h”
using namespace vex;
motor Motor1(PORT1, ratio18_1, false);
int main() {
Motor1.spin(forward, 50, percent);
return 0;
}
Using Loops for Speed Control
Iterative Adjustments
Loops let you progressively either raise or lower motor speed:
cpp
Copy code
for (int speed = 0; speed <= 100; speed += 10) {
Motor1.spin(forward, speed, percent);
wait(1, seconds);
}
Controlling Multiple Motors Simultaneously
Synchronizing Motors
Driving straight depends on synchronising motors for jobs like these. Here is how:
cpp
Copy code
motor LeftMotor(PORT1, ratio18_1, false);
motor RightMotor(PORT2, ratio18_1, true);
LeftMotor.spin(forward, 50, percent);
RightMotor.spin(forward, 50, percent);
Incorporating Sensors for Speed Control
Dynamic Adjustments with Sensors
Distance sensors among other types of sensors allow motor speed to be automatically changed:
cpp
Copy code
distance DistanceSensor(PORT3);
if (DistanceSensor.objectDistance(mm) < 200) {
Motor1.spin(forward, 30, percent);
} else {
Motor1.spin(forward, 70, percent);
}
Advanced Techniques: PID Control
What Is PID?
PID (Proportional, Integral, Derivative) control keeps constant speed independent of changing load. Using it means computing error and dynamically changing motor power.
Debugging Motor Speed Issues
Common Errors
- Incorrect motor port assignments.
- Conflicting commands.
Troubleshooting Tips
Track motor performance in real time with VEXcode’s built-in Debugger.
What is the Default Speed of a VEX V5 Motor?
If you have ever worked with robotics, VEX V5 motors most likely come across. In the realm of competitive and instructional robotics, these motors are a powerhouse. Still, one often asked question is, “What is the default speed of a VEX V5 motor?”Let us address this topic, analyze the specifics, and look at how this information influences useful applications.
Overview of VEX V5 Motors
Types of VEX V5 Motors
Although set motor speed c vex v5 provides a selection of motors meant for different uses, the V5 Smart Motor takes front stage. Its simplicity of use, accuracy, and efficiency are well known.
Key Specifications of VEX V5 Motors
- Voltage: Operates on a nominal 12V
- Torque: Adjustable to fit different tasks
- Built-in Sensors: For programming changes, offer real-time comments.
Default Speed Settings
What Does “Default Speed” Mean?
The default speed is the factory-set speed the motor runs at in case no particular hardware or programming changes are made.
The Default Speed of VEX V5 Motors
Standard speed of the VEX V5 Smart Motor is 100 revolutions per minute (RPM). This speed is ideal for a range of jobs right out of the box since it strikes a mix between torque and efficiency.
Conclusion
For those who enjoy robotics, knowing how to set motor speed in C for VEX V5 is fundamental ability. Once you have this under control, you open limitless creative and innovative opportunities. Dive in, play about, and see how your thoughts come to pass.
FAQs
- What is the default speed of a VEX V5 motor?
The default speed is 100% when no specific speed is defined. - Can I control motor speed without programming?
Yes, using the V5 Brain interface manually. - How do I reset motor settings to default?
Disconnect and reconnect the motor to reset its state. - What is the difference between voltage and velocity control?
Voltage control directly adjusts power, while velocity control maintains a consistent speed. - Can I use other programming languages for VEX V5?
Yes, Python is also supported in VEXcode.