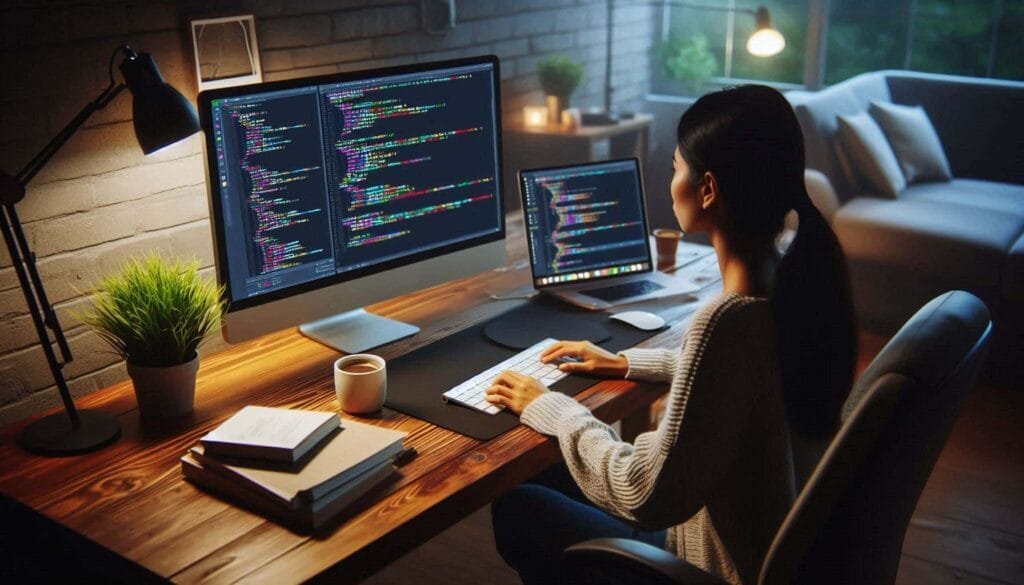
Building effective and useful code in the field of programming depends on knowing the several kinds of commands and structures. Among these, repetitious blocks are rather important. What precisely, though, is a repeat block? The nature of repeat block in code, their uses, and their integration within the larger framework of programming ideas will be discussed in this paper.
1. Introduction to Programming Constructs
Let’s stand back and consider programming structures generally before diving into repeat blocks. Built on several commands and structures that enable developers to manage program flow, programming languages help to One can classify these constructions into numerous groups:
- Sequential Commands: These execute statements one after another.
- Conditional Commands: These allow the program to make decisions based on certain conditions (e.g., if-else statements).
- Iterative Commands: These enable repetition of code, which leads us to repeat blocks.
2. What is a Repeat Block?
An iterative construction, a repeat block lets a certain set of instructions be carried out several times until a given condition is satisfied. In programming, this kind of directive is absolutely vital since it improves code readability and lowers duplicity. A repeat block in code can effectively handle repeated chores rather than having one line of code written over and over.
2.1 Basic Structure of a Repeat Block
The structure of a repeat block varies depending on the programming language, but the concept remains consistent. A typical repeat block consists of:
- Initialization: Setting up any necessary variables.
- Condition: The condition that determines when to stop the repetition.
- Action: The commands or operations that are executed during each iteration.
For example, in pseudocode, a repeat block might look like this:
pseudocode
Copy code
repeat
// Action to perform
until condition
In this structure, the actions inside the block will continue to execute until the specified condition evaluates to true.
3. Types of Repeat Blocks
Repeat blocks can be classified into several types based on their structure and purpose:
3.1 Simple Repeat Blocks
These are the most basic kind of repetitive blocks. They follow a set of directions a predetermined count of times. For five times printing “Hello,” for instance, you may use a basic repeat block.
3.2 Conditional Repeat Blocks
Conditional repetition blocks carry on until a specific criterion is satisfied. Commonly encountered in loops—such as do-while or while loops—where the code will continue running as long as the designated condition is true—
3.3 Counted Repeat Blocks
Counted repeat blocks let one specify a set number of repetitions. Usually, this is carried out via a loop counting down or up to a designated count. For Python, you might count from 1 to 10 using a for loop.
3.4 Infinite Repeat Blocks
These blocks will keep running endlessly as the name suggests until they are manually halted or an exit condition is set into them. This kind of block should be used carefully to prevent infinite loops even if it might be helpful in some cases, such monitoring systems or waiting for user input.
4. Common Programming Languages that Use Repeat Blocks
Many computer languages have as their basic idea repeat blocks. Here are a few instances of how various languages apply repeat blocks:
4.1 Python
In Python, the while and for loops serve as repeat blocks. Here’s an example using a while loop:
python
Copy code
count = 0
while count < 5:
print(“Hello”)
count += 1
4.2 Java
Java uses for, while, and do-while loops as repeat blocks. For instance:
java
Copy code
for (int i = 0; i < 5; i++) {
System.out.println(“Hello”);
}
4.3 JavaScript
In JavaScript, similar constructs exist, allowing for easy implementation of repeat blocks:
javascript
Copy code
let count = 0;
while (count < 5) {
console.log(“Hello”);
count++;
}
4.4 Scratch
Often used as a visual programming language for teaching coding, Scratch makes use of visually controllable repeat blocks. Dragging a repeat block allows a user to indicate how many times they wish to do the enclosing activities.
5. Benefits of Using Repeat Blocks
Repeat blocks offer numerous advantages in programming:
5.1 Reducing Code Redundancy
Repeat blocks let developers create more effective and neat code. This not only makes reading the code simpler but also lowers the possibility of mistakes resulting from constant typing.
5.2 Improved Maintainability
Having a single repeat block helps to ensure that updates made in one location—rather than scattered around the code—when changes are required.
5.3 Enhanced Performance
Many times, by refining loops and thereby accelerating execution times, repeat blocks can improve the speed of a program.
6. Challenges and Considerations with Repeat Blocks
While repeat blocks are incredibly useful, they also come with their own set of challenges:
6.1 Infinite Loops
Creating infinite loops is one of the most often occurring mistakes in using repeat blocks. This results from a program running eternally since the exit condition is never satisfied.
6.2 Readability Issues
Complicated repeat blocks can make the code difficult to comprehend if not correctly organized or commented upon. Maintaining clarity is crucial, particularly in relation to layering repeated blocks.
6.3 Performance Concerns
Sometimes poorly designed or too complicated repeat blocks cause performance problems. This is particularly true in big projects where effectiveness is absolutely vital.
7. Best Practices for Implementing Repeat Blocks
To get the most out of repeat blocks, consider the following best practices:
7.1 Keep It Simple
Steer clear of excessively complicated repetitive blocks. Whenever at all possible, divide chores into smaller, more doable chunks.
7.2 Use Meaningful Variable Names
Clear variable names help in understanding the purpose of each loop and its conditions.
7.3 Comment Your Code
Comments that clarify the intent of repeat blocks will help you and others much improve code readability.
7.4 Test Thoroughly
Always test your code to ensure that repeat blocks are functioning as intended and not creating infinite loops.
8. Real-World Applications of Repeat Blocks
Repeat blocks have numerous applications in real-world programming scenarios:
8.1 Data Processing
In data analysis, repeat blocks—iteratively across entries—perform computations or transformations—processing vast amounts of data.
8.2 Game Development
Like the game being stopped or ended, game loops can mostly rely on repeat blocks to continuously update the game state until a given condition is satisfied.
8.3 Automation
Repeated blocks in automation scripts might run jobs repeatedly until they are no longer required, say for system monitoring or frequent upgrades.
9. Conclusion
Ultimately, repetition blocks are essential components of programming that enable effective coding and problem-solving. Understanding its structure and use will help developers to maximize their capability to produce strong, maintainable, and effective code. Although repeat blocks have certain difficulties, following optimal standards greatly improves their efficiency.
FAQs
1. What is the difference between a repeat block and a conditional statement?
A repeat block is used for iteration and repetition of code, while a conditional statement is used for decision-making based on certain conditions.
2. Can repeat blocks cause performance issues?
Yes, if not implemented correctly, repeat blocks can lead to performance issues, especially if they create infinite loops.
3. Are repeat blocks used in all programming languages?
While the implementation may vary, the concept of repeat blocks is present in almost all programming languages.
4. How can I avoid infinite loops in repeat blocks?
To avoid infinite loops, ensure that your exit condition is reachable and properly defined.
5. What are some common mistakes when using repeat blocks?
Common mistakes include creating overly complex loops, neglecting to update loop counters, and failing to document the purpose of the loop.